res/layout
folder and name it as webview.xml
and populate it with the following element:<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <WebView android:id="@+id/webview1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text = "Hello, world!" /> </LinearLayout>
src/net.learn2develop.AndroidViews
folder and name it as WebViewExample.java
. Populate it as follows:package net.learn2develop.AndroidViews; import android.app.Activity; import android.os.Bundle; import android.webkit.WebView; public class WebViewExample extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.webview); WebView wv = (WebView) findViewById(R.id.webview1); wv.loadUrl("http://www.mobiforge.com"); } }
loadUrl()
method of the WebView
class loads the page of a given URL. Modify the AndroidManifest.xml
file to register the new activity as well as to request for the INTERNET permission:<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="net.learn2develop.AndroidViews" android:versionCode="1" android:versionName="1.0.0"> <application android:icon="@drawable/icon" android:label="@string/app_name"> <activity android:name=".ViewsActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".WebViewExample" android:label="@string/app_name" /> </application> <uses-permission android:name="android.permission.INTERNET"> </uses-permission> <uses-sdk android:minSdkVersion="3" /> </manifest>
AndroidManifest.xml
file. Modify the ViewsActivity.java
file as follows to start the WebViewExample
activity:package net.learn2develop.AndroidViews; import android.app.Activity; import android.os.Bundle; import android.content.Intent; public class ViewsActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); startActivity(new Intent(this, WebViewExample.class)); } }
WebView
view in action. 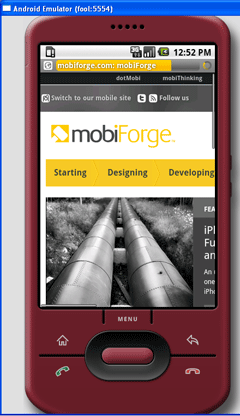
Figure 8 - The WebView displaying a web page
WebView
view, using the loadDataWithBaseURL()
method:WebView wv = (WebView) findViewById(R.id.webview1); final String mimeType = "text/html"; final String encoding = "UTF-8"; String html = "<H1>A simple HTML page</H1><body>" + "<p>The quick brown fox jumps over the lazy dog</p>"; wv.loadDataWithBaseURL("", html, mimeType, encoding, "");
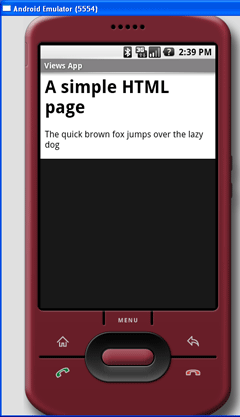
Figure 9 - Displaying the content of a HTML string
Index.html
under the assets folder.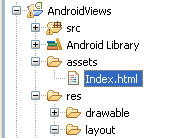
Figure 10 - Adding a HTML page in the assets folder
Index.html
is as follows:<h1>A simple HTML page</h1> <body> <p>The quick brown fox jumps over the lazy dog</p> <img src='http://www.google.com/logos/Logo_60wht.gif'/> </body>
Index.html
file, use the loadUrl()
method as follows:WebView wv = (WebView) findViewById(R.id.webview1); wv.loadUrl("file:///android_asset/Index.html");
WebView
view.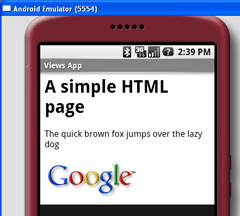
Figure 11 - Loading the HTML page using WebView