onCreateOptionsMenu()
and onOptionsItemSelected()
. The onCreateOptionsMenu()
method is called when the MENU button is pressed. In this event, you would call the CreateMenu()
helper method to display the options menu. When a menu item is selected, the onOptionsItemSelected()
method will be called. In this case, you call the MenuChoice()
method to display the menu item selected (and do whatever you need to do):public class MenuExample extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.menu); } private void CreateMenu(Menu menu) { //... } private boolean MenuChoice(MenuItem item) { //... } //---only created once--- @Override public boolean onCreateOptionsMenu(Menu menu) { super.onCreateOptionsMenu(menu); CreateMenu(menu); return true; } @Override public boolean onOptionsItemSelected(MenuItem item) { return MenuChoice(item); } }
ViewsActivity.java
file as follows to start the MenuExample
activity:package net.learn2develop.AndroidViews; import android.app.Activity; import android.os.Bundle; import android.content.Intent; public class ViewsActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); startActivity(new Intent(this, MenuExample.class)); } }
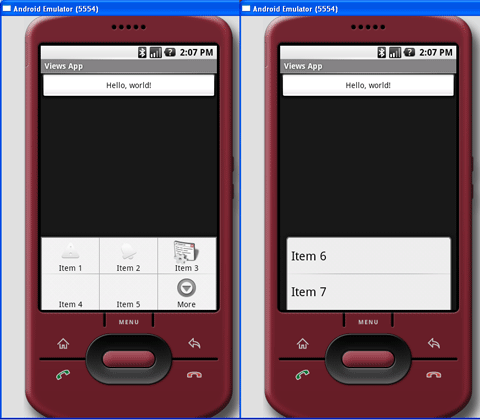
Figure 4 - Displaying a options menu in your application