- Context Menu
- Options Menu
- AnalogClock
- DigitalClock
- WebView
Menus
Menus are useful for displaying additional options that are not directly visible on the main UI of an application. There are two main types of menus in Android:- Context Menu – displays information related to a particular view on an activity. In Android, to activate a context menu you press and hold on to it.
- Options Menu – displays information related to the current activity. In Android, you activate the options menu by pressing the MENU key.
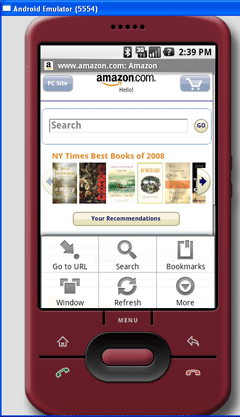
Figure 1 - The options menu in the Browser application
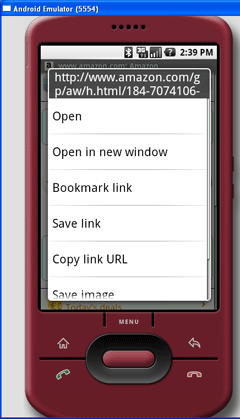
Figure 2 - The context menu in the Browser application
res/layout
folder and name it as menu.xml
. Populate it with the following element:<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <Button android:id="@+id/btn1" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text = "Hello, world!" /> </LinearLayout>
src/net.learn2develop.AndroidViews
folder and name it as MenuExample.java
. You will leave this file alone for now. Modify the AndroidManifest.xml
file to register the new activity:<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="net.learn2develop.AndroidViews" android:versionCode="1" android:versionName="1.0.0"> <application android:icon="@drawable/icon" android:label="@string/app_name"> <activity android:name=".ViewsActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name=".MenuExample" android:label="@string/app_name" /> </application> </manifest>