Using the same activity created in the previous section, insert the following element in the
basicviews.xml
file:<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <ProgressBar android:id="@+id/progressbar" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/btnSave" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Save" /> <!- Other views omitted --> </LinearLayout>
ProgressBar
view is indeterminate - that is, it shows a cyclic animation. This mode is useful for tasks that do not have a clear indication of when they will be completed. For example, you are sending some data to a web service and waiting for the server to respond.The following code shows how you can spin off a background thread to simulate performing some long lasting tasks. When the task is completed, you hide the ProgressBar view by setting its Visibility property to GONE (value 8).
import android.widget.ProgressBar; import android.os.Handler; public class BasicViewsExample extends Activity { private static int progress = 0; private ProgressBar progressBar; private int progressStatus = 0; private Handler handler = new Handler(); @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.basicviews); progressBar = (ProgressBar) findViewById(R.id.progressbar); //---do some work in background thread--- new Thread(new Runnable() { public void run() { //---do some work here--- while (progressStatus < 10) { progressStatus = doSomeWork(); } //---hides the progress bar--- handler.post(new Runnable() { public void run() { //---0 - VISIBLE; 4 - INVISIBLE; 8 - GONE--- progressBar.setVisibility(8); } }); } //---do some long lasting work here--- private int doSomeWork() { try { //---simulate doing some work--- Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } return ++progress; } }).start(); //... //... } //... }
INVISIBLE
and GONE
constants is that the INVISIBLE
constant simply hides the ProgressBar
view. The GONE
constant removes the ProgressBar
view from the activity and does not take up any space on the activity.The left of Figure 5 shows the
ProgressBar
view in action. When the background task is completed, the space taken by the view is given up when you set its Visibility
property to GONE
. 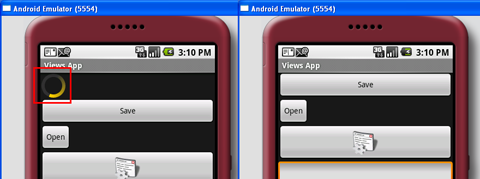
Figure 5 Progressbar view in action (left), disappears when the the background task is completed (right)
ProgressBar
view in indeterminate mode, you can modify it to display as a horizontal bar. The following style attribute specifies the ProgressBar
view to be displayed as a horizontal bar:<ProgressBar android:id="@+id/progressbar"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
/>
ProgressBar
view to increment from 1 to 100 and then disappears off the screen (see also Figure 6):public class BasicViewsExample extends Activity { private static int progress = 0; private ProgressBar progressBar; private int progressStatus = 0; private Handler handler = new Handler(); @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.basicviews); progressBar = (ProgressBar) findViewById(R.id.progressbar); //---do some work in background thread--- new Thread(new Runnable() { public void run() { while (progressStatus < 100) { progressStatus = doSomeWork(); //---Update the progress bar--- handler.post(new Runnable() { public void run() { progressBar.setProgress(progressStatus); } }); } //---hides the progress bar--- handler.post(new Runnable() { public void run() { //---0 - VISIBLE; 4 - INVISIBLE; 8 - GONE--- progressBar.setVisibility(8); } }); } private int doSomeWork() { try { //---simulate doing some work--- Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } return ++progress; } }).start(); //... //... } }
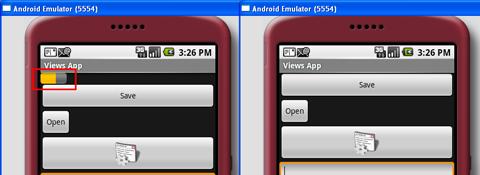
Figure 6 Horizontal Progressbar view in action (left), disappears when the the background task is completed (right)